+-
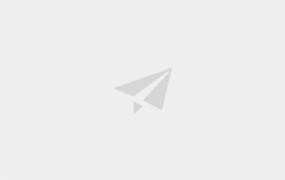
简介
Axios 是一个基于 Promise 的 HTTP 客户端,同时支持浏览器和 Node.js 环境。它是一个优秀的 HTTP 客户端,被广泛地应用在大量的 Web 项目中。具体介绍可见官方文档
对于大多数应用来说,都会遇到要统一处理ajax请求的场景,为了较好地解决这个问题,拦截器就应运而生了。
在Axios中它提供了请求拦截器和响应拦截器来分别处理请求和响应,它们的作用如下:
接下来,本文将通过axios源码来阐述拦截器是如何设计实现的。
设计与实现
任务的注册
首先以下面的代码为例,通过use将方法注册到拦截器中
// request interceptor
axios.interceptors.request.use(
config => {
console.log('config', config);
return config;
},
err => Promise.reject(err),
);
// response interceptor
axios.interceptors.response.use(response => {
console.log('response', response);
return response;
});
// axios/lib/core/InterceptorManager.js
// 在拦截器管理类中通过use方法向任务列表中添加任务
InterceptorManager.prototype.use = function use(fulfilled, rejected) {
this.handlers.push({
fulfilled: fulfilled,
rejected: rejected
});
return this.handlers.length - 1;
};
任务的编排
// 先看lib/axios.js(入口文件) 中的创建实例的方法
function createInstance(defaultConfig) {
var context = new Axios(defaultConfig);
// REVIEW[epic=interceptors,seq=0] 在axios对象上绑定request方法,使得axios({option})这样的方式即可调用request方法
var instance = bind(Axios.prototype.request, context);
// Copy axios.prototype to instance
utils.extend(instance, Axios.prototype, context);
// Copy context to instance
utils.extend(instance, context);
return instance;
}
// 重点在于Axios.prototype.request
Axios.prototype.request = function request(config) {
// ...已省略部分代码
// Hook up interceptors middleware
// REVIEW[epic=interceptors,seq=2] dispatchRequest 为我们使用axios时,项目中调用的请求
var chain = [dispatchRequest, undefined];
var promise = Promise.resolve(config);
// REVIEW[epic=interceptors,seq=4] 向拦截器任务列表的头部注册 request任务
this.interceptors.request.forEach(function unshiftRequestInterceptors(interceptor) {
chain.unshift(interceptor.fulfilled, interceptor.rejected);
});
// REVIEW[epic=interceptors,seq=5] 向拦截器任务列表的尾部注册 response任务
this.interceptors.response.forEach(function pushResponseInterceptors(interceptor) {
chain.push(interceptor.fulfilled, interceptor.rejected);
});
// 通过上面的注册方式,我们可以知道最后的chain数组会长成的样子是:
// [ ...requestInterceptor, dispatchRequest,undefined, ...responseInterceptor ]
// 这样就保证了拦截器执行的顺序
while (chain.length) {
// 因为是成对注册的任务(fulfilled, rejected)所以执行的时候也是shift2次
promise = promise.then(chain.shift(), chain.shift());
}
return promise;
};
可以看出通过从不同的位置向任务列表中添加任务,实现了任务的编排,达到了按requestInterceptor => Request => responseInterceptor 顺序执行的目的
任务的调度
任务的调度主要是看上面 request函数中的这一行
var promise = Promise.resolve(config);
while (chain.length) {
// 因为是成对注册的任务(fulfilled, rejected)所以执行的时候也是shift2次
promise = promise.then(chain.shift(), chain.shift());
}
可以看出就是按注册的顺序依次执行,并且每个任务中都需要返回config。
结语
在这次的源码阅读时,明显感觉到,因为之前几次的积累,读源码这件事开始变得没有那么的“困难”了。但是在写文档的时候如何更清晰地表达,还是遇到了点问题。因此借鉴了下网上已有的文档,使用了任务注册 => 任务编排 => 任务调度,以任务为视角来做解析的方式来阐述代码。